1. Introduction
In the realm of modern web development, creating dynamic and interactive applications is essential to meet the demands of users. Angular, a popular front-end framework maintained by Google, provides developers with a robust set of tools and features to build such applications efficiently. One common requirement in web development is the need for CRUD operations – Create, Read, Update, and Delete – to manage data within an application.
In this guide, we will embark on a journey to develop a CRUD (Create, Read, Update, Delete) product application using Angular14, Nx, Material UI, and RxJS. We’ll leverage Angular’s powerful capabilities to create a seamless user experience for managing products within our application.
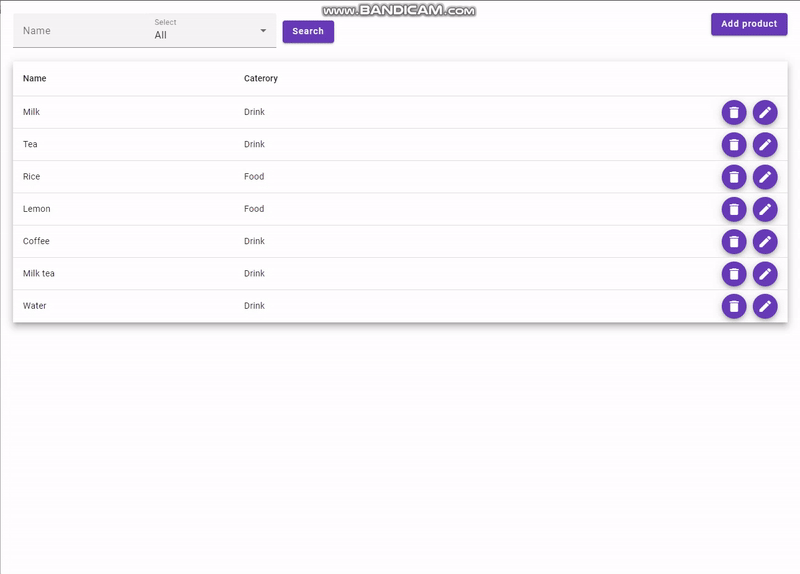
2. How the app works?
Our app implements CRUD operators for the product data of a company, the product schema is:
- Id: id of product
- CompanyId: the company owns this product
- Name: product name
- Category: product’s category (one of the predefined categories: “Drink”, “Food”, “Electronic “, “Book”)
Below is the initial product data which belongs to two companies (companyId= 1 and companyId= 2)
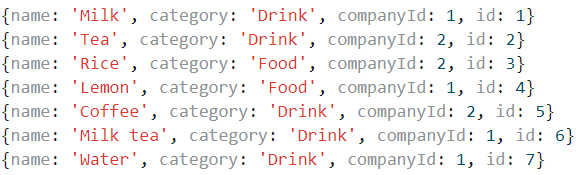
The app contains only one screen – product management accessed via the URL: http://localhost:4200/company/{companyId}/products, the CRUD functions and corresponding backend API are:
GET /company/{companyId}/products?category={category}&name={name} | Load products by company id, name and category |
POST /company/{companyId}/products | Create new product |
PUT /company/{companyId}/products/{id} | Update a product |
DELETE /company/{companyId}/products/{id} | Delete a product |
GET /company/{companyId}/categories | Get categories |
3. Tech stack
- Nx: manage and build Angular app (learn more)
- Material UI: reusable UI component (learn more)
- RxJS: handle asynchronous operations, we will use it to call backend API
- Docker: start backend API (I built this mock API in order to demostrate this article, the Docker image is publish on my Docker hub)
Majority of our project contains 3 components and a service to interact with backend API as below.
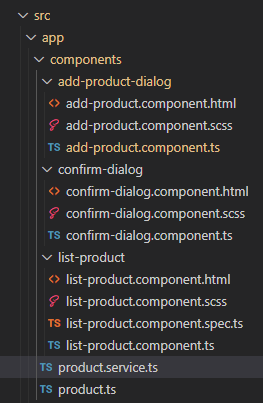
4. Using RxJS to call RESTful API
Before creating some components to display product data, we need to create a service to interact with backend API using RxJS.
product.service.ts
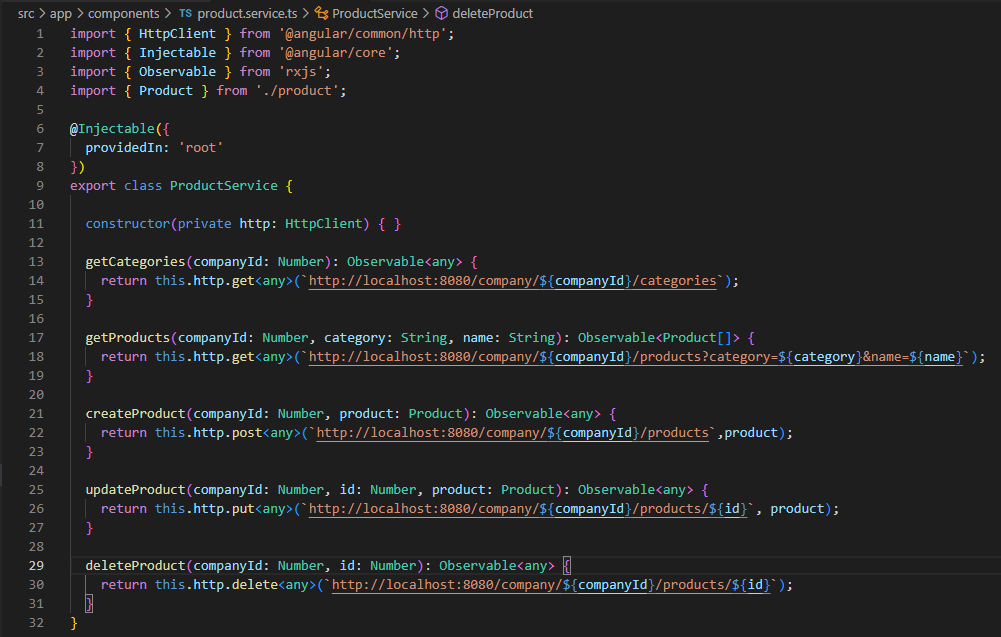
5. Components design
5.1. List product component
This component takes responsibility for loading and displaying product table based on companyId on the URL and supports searching products by name and category. We use ActivatedRoute to extract companyId from the URL, after that we will call backend API to:
- Load categories from companyId
- Load all products from companyId. Not that the loadProducts method requires three parameters: companyId, category, productName. At this step, we will load all products of the company by passing productName = ” and selectedCategory = ”
list-product.component.ts
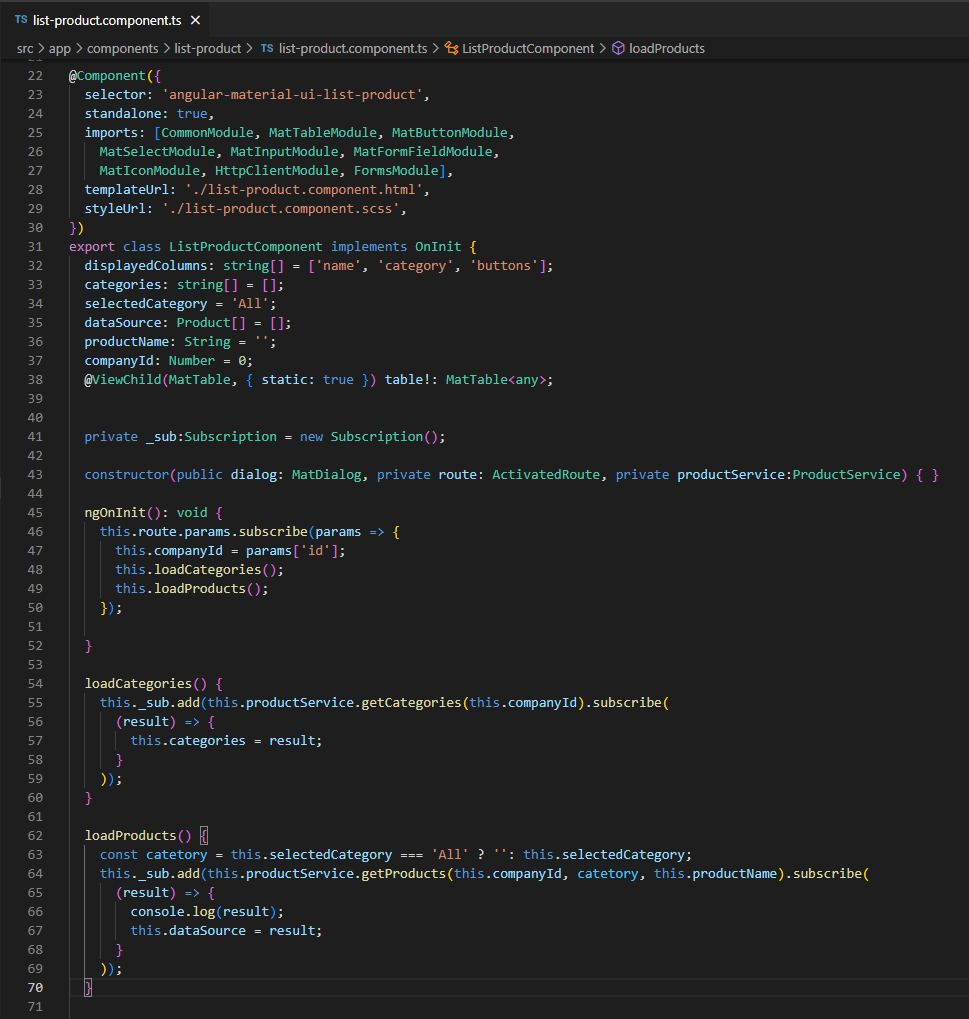
list-product.component.html
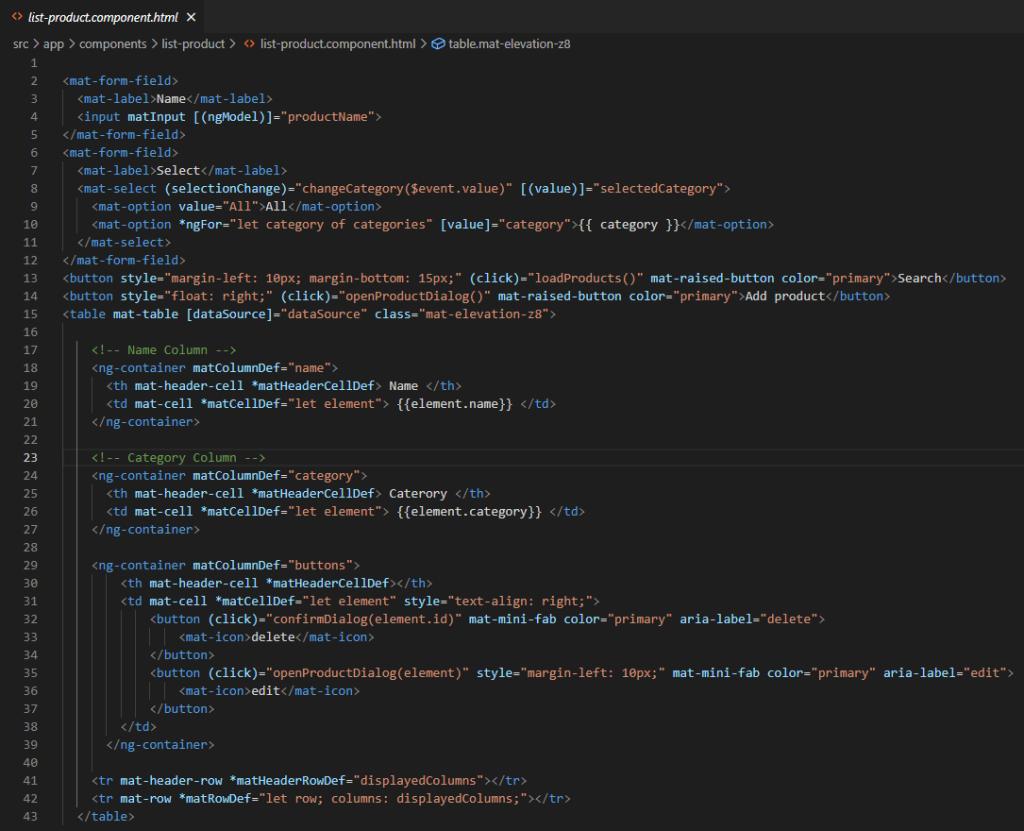
To implement the search product function, we create two variables productName and selectedCategory then bind them to the input and select-option on HTML. After that, when the user hits the “Search” button we just need to call the loadProducts() function to perform the search.
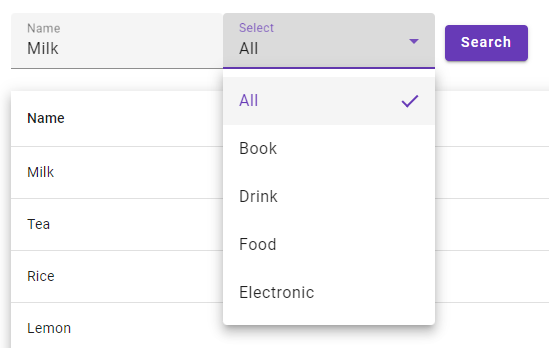
5.2. Add product component
This component is used for both create and edit product functions via a dialog as below.
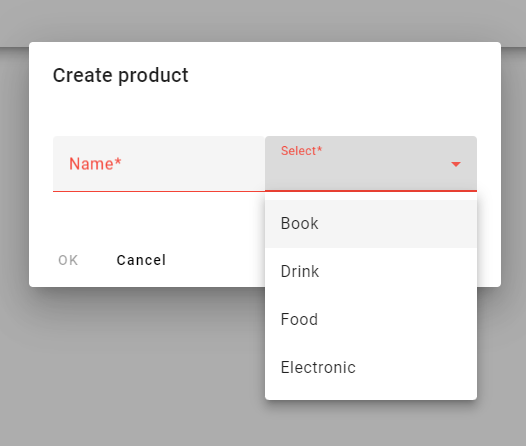
We will build this component based on Material Dialog, bascically the add-product component consumes some data passed to MAT_DIALOG_DATA:
- categories: to build the category drop-down
- product: to display product info for the update product function
We will use Angular Reactive forms which is a model-driven approach to handling form inputs whose values change over time to build the product form containing two fields: product name and category
add-product.component.ts
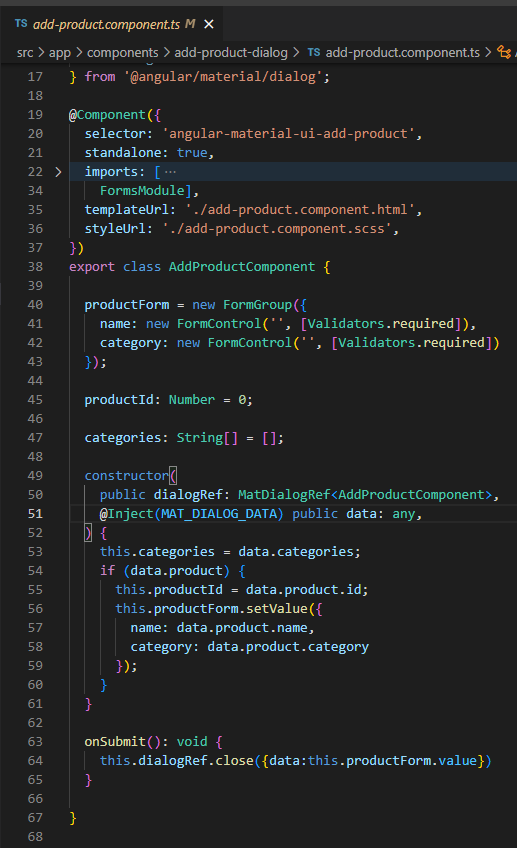
add-product.component.html
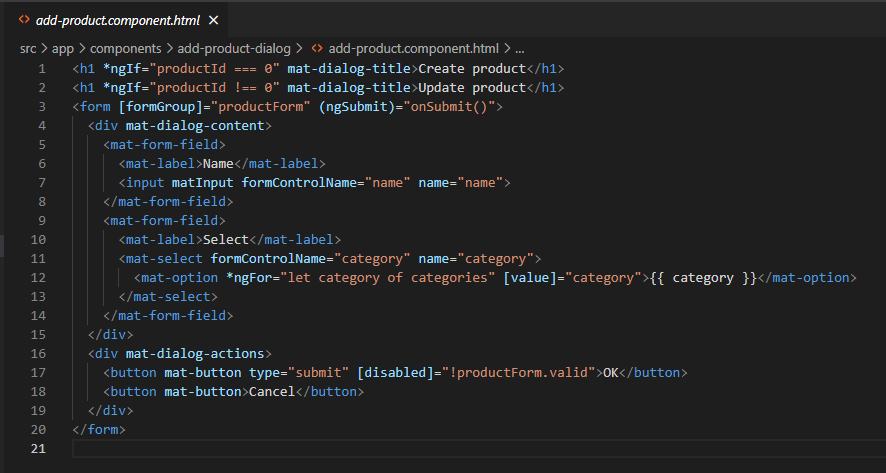
On the list product component, we create a openProductDialog method with product parameter to open the addProduct dialog and perform the create/update product respectively:
- When the user hits the update product button we will pass the selected product to this method
- when hitting the create product button we won’t pass any argument to this method
list-product.component.ts
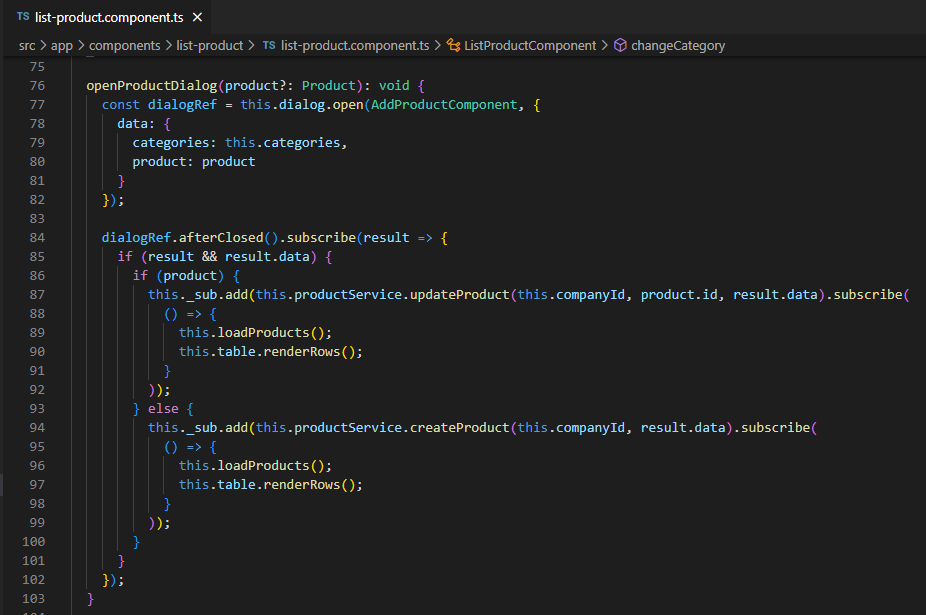
5.3. Delete product component
We will create a reusable confirm dialog to implement the delete function. When hitting Yes the DELETE product API will be called.
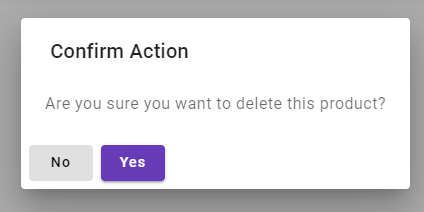
confirm-dialog.component.ts
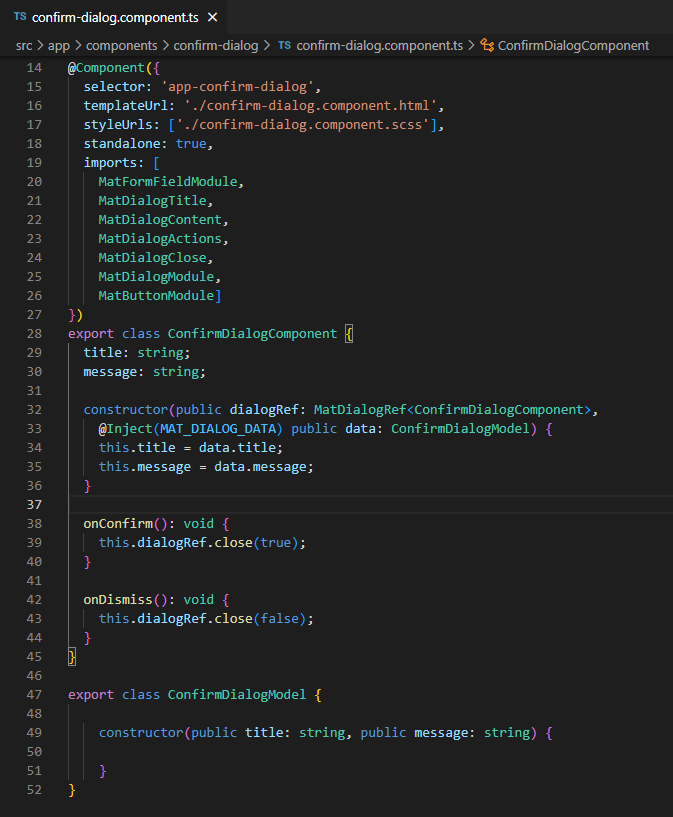
list-product.component.ts
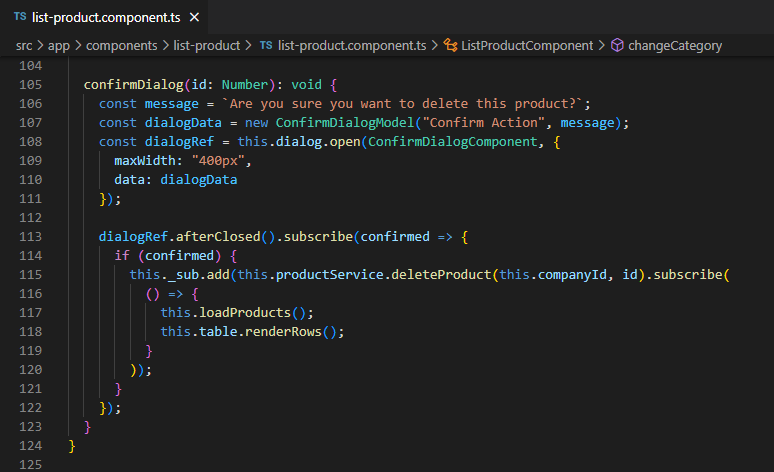
6. Conclusion
Building a CRUD application with Angular involves leveraging the framework’s powerful features, including components, services, routing, and RxJS. Through this article, we gain practical experience in creating a full-fledged web application that interacts with a backend server to perform basic CRUD operations on data.
By mastering Angular’s concepts and leveraging its features effectively, developers can build dynamic and interactive user interfaces that deliver a seamless user experience. This demo application can be found on GitHub.